Exercise: Powernap
Powernap is a simple Peer-to-peer file sharing system, inspired by Napster.
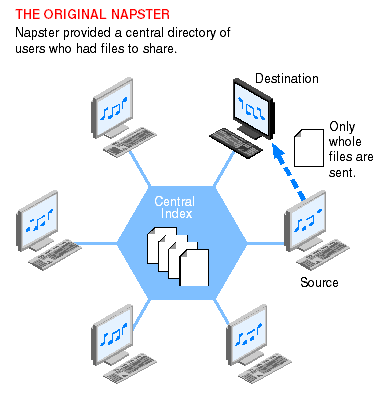
The central Index (aka. Registry aka. Repository) keeps track of the addresses (host, port) of each file in the system.
The files are not in the Index - they are on one (or more) peers.
In this exercise you must program the Index and the Peer, using mixed architecures and techonolgies:
Application types and communication
- The Index must be a SOAP based WCF web service hosted in Azure
- The Peer must be an ordinary application (maybe as simple as Console Application)
Communication between peers using ordinary socket API.
Architectures
- The Peer and the Index has a client/server relationship
Peer = client, Index = server
- The Peers has a Peer-to-Peer relationship
Each peer plays the role of a client AND a server.
Organization: Alone or together
You can do this exercise alone or together with ONE other programmer.
If you decide to do it with another programmer I suggest you do the following:
- Together you define the interface part of the WCF service Index
- One programmer implements the WCF service
- The other programmer makes the Peer.
Index aka. Registry aka. Repository
The Index must be developed as a Web Service hosted in Microsoft Azure.
The web service must be backed by some kind of storage, like a relational database.
For each file we want to store (filename, host, port).
The same filename can be present on many (host, port).
However, a given (filename, host, port) can appear at most once in the storage.
Getting ready
Make a new Visual Studio project (type WCF -> WCF Service Application) named RepositorySoapService (or something similar).
Web service interface
Define the web service interface, i.e. the interface in the newly created project.
Which operations do you need?
- Count()
Counts all the entries in the index. Useful for testing ...
select count(filename)
- CountFilenames()
Counts all different filenames in the index.
select count(distinct filename)
- bool Add(filename, host, port)
returns true if something was added to the index.
- List<Destination> Get(filename)
Destination is a class [DataContract] with properties host + port.
- bool Remove(filename, host, port)
returns true if something was removed from the index.
- Extra: int howMany AddAll(List<filename>, host, port)
- Extra: int howMany RemoveAll(host, port)
Storage creation
Create some kind of storage in Microsoft Azure - maybe a relational database, maybe another kind of storage - to support your web interface.
Web service implementation
Implement the web service: The methods should be implemented one-by-one.
- Implement one method
- Run the web service locally
- Try the web service using WcfTestClient
- Unit test the method
Peer
Program the peer as a WPF simple application. The user interface might look as ugly as this
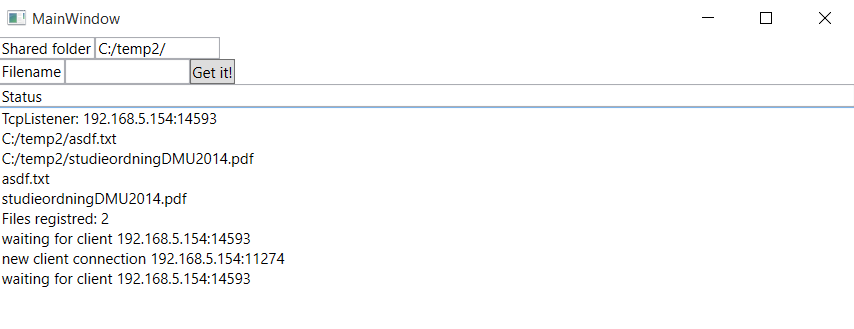
My user interface is define in the XAML file MainWindow.xaml.
Start up (peer joins the "network"):
- Peer connects to the Index and Add all it's files (from the "Shared folder") to the Index
string files = Directory.GetFiles(...); might be useful
The web service method AddAll(...) might be useful.
Requesting a file
- Peer (client part) connect to the Index and gets information on the locations of the file
The web service method Get(...) might be useful.
- The peer (client part) chooses on the th locations and connects to another peer (server part)
and sends a request for the file.
- The other peer (server part) sends back the reqested file to this peer (client part).
This peer (client part) saves the file in the "Shared folder".
Maybe we need to add a little protocol here to be able to tell the client part of the file existed or not.
Inspiration from the HTTP protocol: First line of the response could be "200 OK"
or "404 File not found".
The file should exist (at least the registry says so ...), but what if it does not?
Shut down (peer leaves the "network"), usually because the user shuts down the application
- Peer connects to the Index and Remove all it's files from the Index
The web service method RemoveAll(...) might be useful.
You can execute code as the Main Windows shuts down http://stackoverflow.com/questions/10018308/execute-code-when-a-wpf-closes
Extra: Peer as Windows Store application (or some other kind of application ...)
Program the Peer again, this time as a Windows Store application - to see which network related API's your are (not) allowed to use.